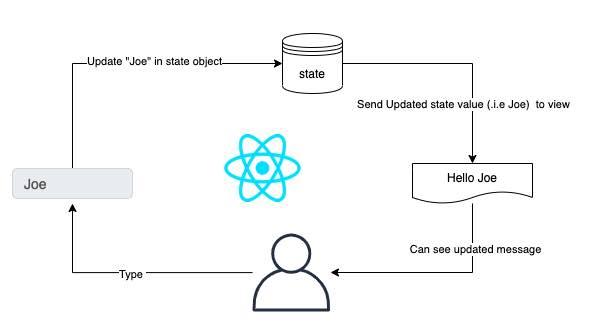
In ReactJS, each component has two aspects: one is view and second is content or data. View part is defined by using JSX or HTML component and data is stored in variables. To hold the data inside a component, you have three options.
- Variable
- Props
- And state
Here , if you put something in variable, you can use these values until component gets rendered, after the component is visible to user and then you change value of that variable, it does not get reflected in the view.
If you use props, again, the props are assigned just before component gets rendered and you cannot change values held in props after that. Its immutable value which is passed to component as argument when you call them to render.
Now, state is the option where you can put the value at initial state, and you can update the value even after the component is visible. Whenever you update value in state using setState() function, component gets rendered again automatically.
Here is the example in which you type something in text box, this value gets updated in state and then the value gets rendered on view automatically.
import React from 'react';
import ReactDOM from 'react-dom';
class SayHello extends React.Component{
constructor(props){
super();
this.state={
name:"Unknown"
}
}
setName=(nameValue)=>{
this.setState({name:nameValue});
}
render(){
return (
<div>
<h1>Hello {this.state.name}</h1>
<input type="text" onChange={e=>this.setName(e.target.value)}/>
</div>
);
}
}
ReactDOM.render(
<React.StrictMode>
<SayHello/>
</React.StrictMode>,
document.getElementById('root')
);