This Transformer converts the json string into java object. You can specify the type of object as well. Here is the example for using the json to object transformer.
- Go to the menu File -> New -> Mule Project and Create the Project demo1
- from the Mule Palette, add the HTTP component and in the general tab of the HTTP component, provide the following configurations
- In General setting Provide the connection configuration. If it does not exists, add new Connector configuration by clicking on + button.
- In Basic Settings, Provide the Path "/form" and allowed method POST or GET
- Add the Message Transformer JSON to Object from Mule Palette.
- In the setting of the JSON to Object Transformer, in General setting, provide the Return Class. This transformer will return the object type of the provided class, for example, provide here java.util.HashMap.
- Add the component Set Payload and in the settings, add the value in which we are putting the payload in message. If the payload would be the type of HashMap , it will return the string form of this object produced by toString() method of HashMap.
- In Postman, create the POST request for the url localhost:8081/form.
- Add the raw body for this request and add the json data {"email":"josnData@sdfs.com","password":"23423423","name":"Test Name","dob":"2018-4-4"}
Using Custom Object in JSON To Object Transformer
The above example converts the received data in HashMap object. Sometimes we need the data in our custom object like User object to process it further. This Transformer able to produce the object of the custom class having the data received in JSON string. The received JSON object properties are populated automatically to the properties of the newly created object. If any property name of the JSON object does not match the property of the object, it throws the mapping exception and asks to annotate that property to be ignorable.
Suppose you want to map the JSON data to custom java class User in above example, User can do it by specifying that class in return class setting of the JSON to object transformer component.
package demo2;
import java.io.Serializable;
import org.codehaus.jackson.annotate.JsonIgnoreProperties;
public class User implements Serializable{
private String username;
private String password;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Override
public String toString() {
return "User [username=" + username + ", password=" + password + "]";
}
}
Here is the flow xml file
<?xml version="1.0" encoding="UTF-8"?>
<mule xmlns:json="http://www.mulesoft.org/schema/mule/json" xmlns:http="http://www.mulesoft.org/schema/mule/http" xmlns="http://www.mulesoft.org/schema/mule/core" xmlns:doc="http://www.mulesoft.org/schema/mule/documentation"
xmlns:spring="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-current.xsd
http://www.mulesoft.org/schema/mule/core http://www.mulesoft.org/schema/mule/core/current/mule.xsd
http://www.mulesoft.org/schema/mule/http http://www.mulesoft.org/schema/mule/http/current/mule-http.xsd
http://www.mulesoft.org/schema/mule/json http://www.mulesoft.org/schema/mule/json/current/mule-json.xsd">
<http:listener-config name="HTTP_Listener_Configuration" host="0.0.0.0" port="8081" doc:name="HTTP Listener Configuration"/>
<flow name="demo2Flow">
<http:listener config-ref="HTTP_Listener_Configuration" path="/demo1" allowedMethods="POST" doc:name="HTTP"/>
<logger message="1 - #[payload]" level="INFO" doc:name="Logger"/>
<json:json-to-object-transformer doc:name="JSON to Object" returnClass="demo2.User"/>
<logger message="2 - #[payload]" level="INFO" doc:name="Logger"/>
<set-payload value="Welcome #[payload.username]" doc:name="Set Payload"/>
</flow>
</mule>
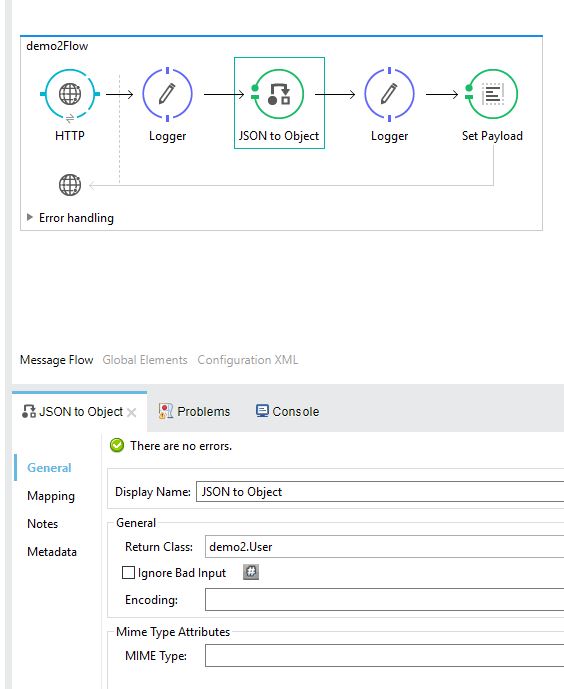
Postman Request