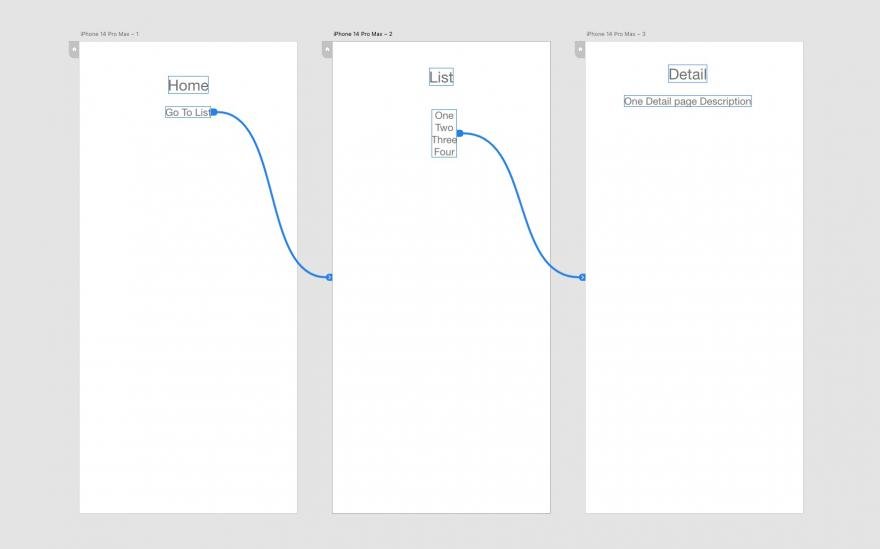
In the Mobile app, we need to navigate between different screens, and each platform has its way of implementation. But in react native, we use the same code base for building a mobile app for all platforms.
Fortunately, you don't need to write the platform-specific code for creating navigation; it has already been done by someone. We have react-navigation library, which creates navigation for us and handles underlying platform complexities.
Here is a simple example.
Detail Component
import React from 'react';
import {View,Text} from "react-native";
export default function Detail(props) {
return (<View>
<View><Text style={{fontSize: 28}}>{props.route.params.detail.title}</Text></View>
<View><Text style={{fontSize: 20}}>{props.route.params.detail.description}</Text></View>
</View>);
}
List component
import React from 'react';
import {View, Text, Pressable} from "react-native";
import Detail from "./detail";
const jsonDta=[
{
title:"One",
description:"One Description value"
},
{
title:"Two",
description:"Two Description value"
},
{
title:"Three",
description:"Three Description value"
},
{
title:"Four",
description:"Four Description value"
},
];
export default function List(props) {
const listing=jsonDta.map(d=> {return <View key={d.title} >
<Pressable onPress={()=>props.navigation.navigate('Detail',{detail:d})}>
<Text style={{fontSize: 18}}>{d.title}</Text>
</Pressable>
</View>});
return (<View>
<View><Text style={{fontSize: 28}}>Listing</Text></View>
{listing}
</View>);
}
Home Component
import React, {useEffect, useState} from 'react';
import {View, Text, Pressable} from "react-native";
export default function Home(props) {
return (<View>
<View><Text style={{fontSize: 28}}>Home</Text></View>
<View><Text style={{fontSize: 14}}>This is Home page body.</Text></View>
<Pressable onPress={()=>props.navigation.navigate('List')}>
<Text>Go to List</Text>
</Pressable>
</View>);
}
App Component
import Home from "./components/home";
import { NavigationContainer } from '@react-navigation/native';
import {createNativeStackNavigator} from "@react-navigation/native-stack";
import List from "./components/list";
import Detail from "./components/detail";
const MainStack=createNativeStackNavigator();
export default function App() {
return (
<NavigationContainer>
<MainStack.Navigator>
<MainStack.Screen
name="Home"
component={Home}
options={{title: 'Home Screen'}}
/>
<MainStack.Screen
name="List"
component={List}
options={{title: 'List'}}
/>
<MainStack.Screen
name="Detail"
component={Detail}
options={{title: 'Detail'}}
/>
</MainStack.Navigator>
</NavigationContainer>
);
}