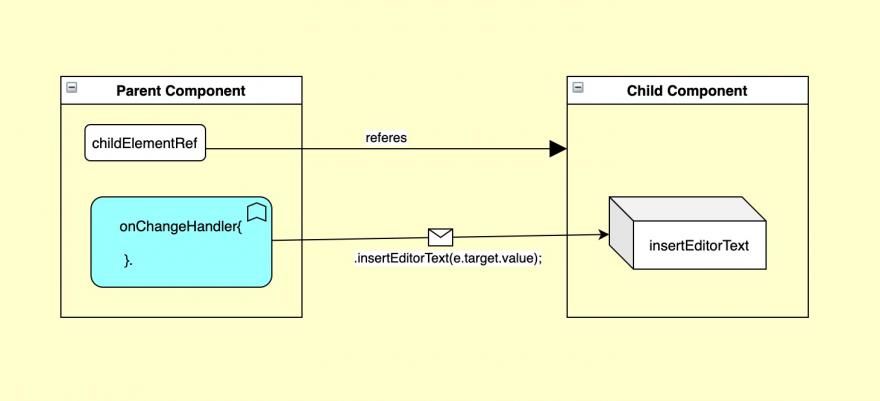
In reactjs, Using forwardRef , you can forward reference of current react component to the component which would use this. And that component can store the returned reference to its local variable and then can access functions or properties of the component(which forwarded reference).
import {useState,forwardRef, useImperativeHandle} from 'react';
const ChildElement = (props,ref) => {
const [state, setState] = useState();
useImperativeHandle(ref, () => ({
insertEditorText (text) {
setState(text);
}
}), [state]);
return (
<input type="text" value={state}/>
);
}
export default forwardRef(ChildElement);
Parent component which would call the function insertEditorText from child element.
import {useState, useEffect, useRef} from 'react';
import ChildElement from 'ChildElement';
const ParentComponent = (props) =>{
const childElementRef = useRef();
const onChangeHandler=(e)=>{
childElementRef.current.insertEditorText(e.target.value);
}
return(
<div>
Parent Input : <input type="text" onChange={onChangeHandler}/>
Child Element: <ChildElement ref={ChildElement}/>
</div>
)
}
Here, you would type in parent text field, and it will get reflected in child component's input field.